In the world of DIY electronics, combining sensors with microcontrollers opens up a realm of possibilities for monitoring and measuring various environmental factors. One such project involves using an NTC 10K thermistor (Viessmann Speichertemperatursensor NTC 10k l=3750 7725998) and an ESP32 microcontroller to measure water temperature accurately. In this guide, we’ll walk you through the process of setting up this simple yet effective temperature monitoring system.
Materials Needed
- ESP32 microcontroller
- NTC 10K thermistor (e.g.: Viessmann Speichertemperatursensor NTC 10k l=3750 7725998)
- Resistor (10K ohms)
- Breadboard and jumper wires
- Voltage regulator (optional, if required for your ESP32 module)
- Power source (e.g., USB power supply)
Understanding the NTC 10K Thermistor (Viessmann Speichertemperatursensor NTC 10k l=3750 7725998):
An NTC (Negative Temperature Coefficient) thermistor is a type of temperature-sensitive resistor whose resistance decreases as the temperature rises. The NTC 10K thermistor (Viessmann Speichertemperatursensor NTC 10k l=3750 7725998) is specifically designed to have a resistance of 10,000 ohms at 25 degrees Celsius.
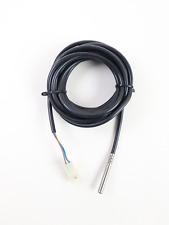
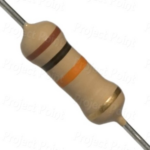
Wiring the Circuit
- Connect one end of the NTC thermistor to the 3.3V output on the ESP32.
- Connect the other end of the NTC thermistor to the analog pin on the ESP32.
- Connect one end of the 10K-ohm resistor to the same analog pin on the ESP32.
- Connect the other end of the 10K-ohm resistor to the ground (GND) pin on the ESP32.
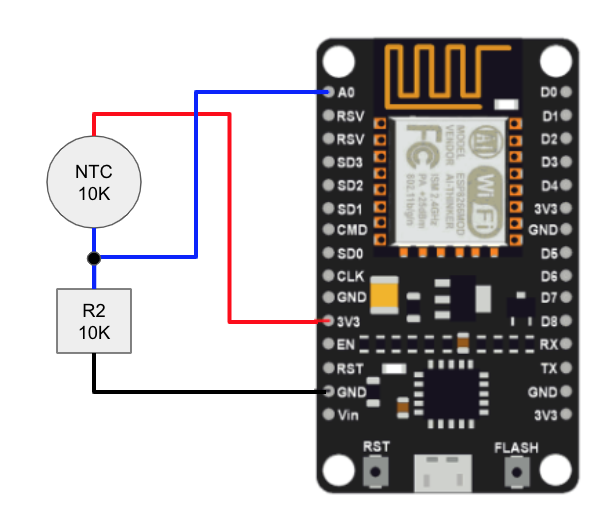
Coding the ESP32: To make the ESP32 read the temperature from the NTC thermistor, you’ll need to write a simple Arduino sketch. Here’s an example code snippet:
const int analogPin = 34; // Analog pin connected to the NTC thermistor
const int resistorValue = 10000; // Resistor value in ohms
void setup() {
Serial.begin(115200);
}
void loop() {
int sensorValue = analogRead(analogPin);
// Convert analog reading to resistance
float resistance = resistorValue / ((1023.0 / sensorValue) - 1.0);
// Use the Steinhart-Hart equation to convert resistance to temperature in Celsius
float temperature = log(resistance / 10000.0) / 3950.0 + 1.0 / (25 + 273.15);
// Convert temperature to Fahrenheit if desired
float temperatureF = (temperature * 9.0 / 5.0) + 32.0;
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C | ");
Serial.print(temperatureF);
Serial.println(" °F");
delay(1000); // Delay for one second before reading again
}
Understanding the Code
- The analogRead function reads the analog value from the NTC thermistor.
- The obtained analog value is then converted to resistance using the known resistor value.
- The Steinhart-Hart equation is used to convert the resistance to temperature in Celsius.
- Optionally, the temperature can be converted to Fahrenheit.
- The temperature readings are then printed to the serial monitor.
A simpler implementation variant is to use the out of the box termistor.h library, as it is shown below:
#include <Thermistor.h>
#include <NTC_Thermistor.h>
#define Referenzwiderstand 10000 // Widerstandswert des Widerstandes der mit dem NTC in Reihe geschaltet wurde.
#define Nominalwiderstand 10000 // Widerstand des NTC bei Normaltemperatur
#define Nominaltemperatur 25 // Temperatur, bei der der NTC den angegebenen Widerstand hat
#define BWert 3750 // Beta Koeffizient(zu finden im Datenblatt des NTC)
Thermistor* thermistor;
// Water temperatur Viessmann NTC 10K Termistor Sensor
// Needs to connect to one of the ESP32 or ESP8866 analog input pins like A0
int TERMISTORPIN = A0;
// init termistor
thermistor = new NTC_Thermistor(TERMISTORPIN, Referenzwiderstand, Nominalwiderstand, Nominaltemperatur, BWert);
// Read water temperature
const double celsius = thermistor->readCelsius();
Conclusion
By combining the power of an NTC 10K thermistor with an ESP32 microcontroller, you can create a reliable water temperature monitoring system for various applications. This project provides an excellent introduction to sensor interfacing and analog signal processing using popular DIY electronics components. Experiment with different resistor values or sensor placements to fine-tune your setup for optimal accuracy in measuring water temperature. Happy tinkering!